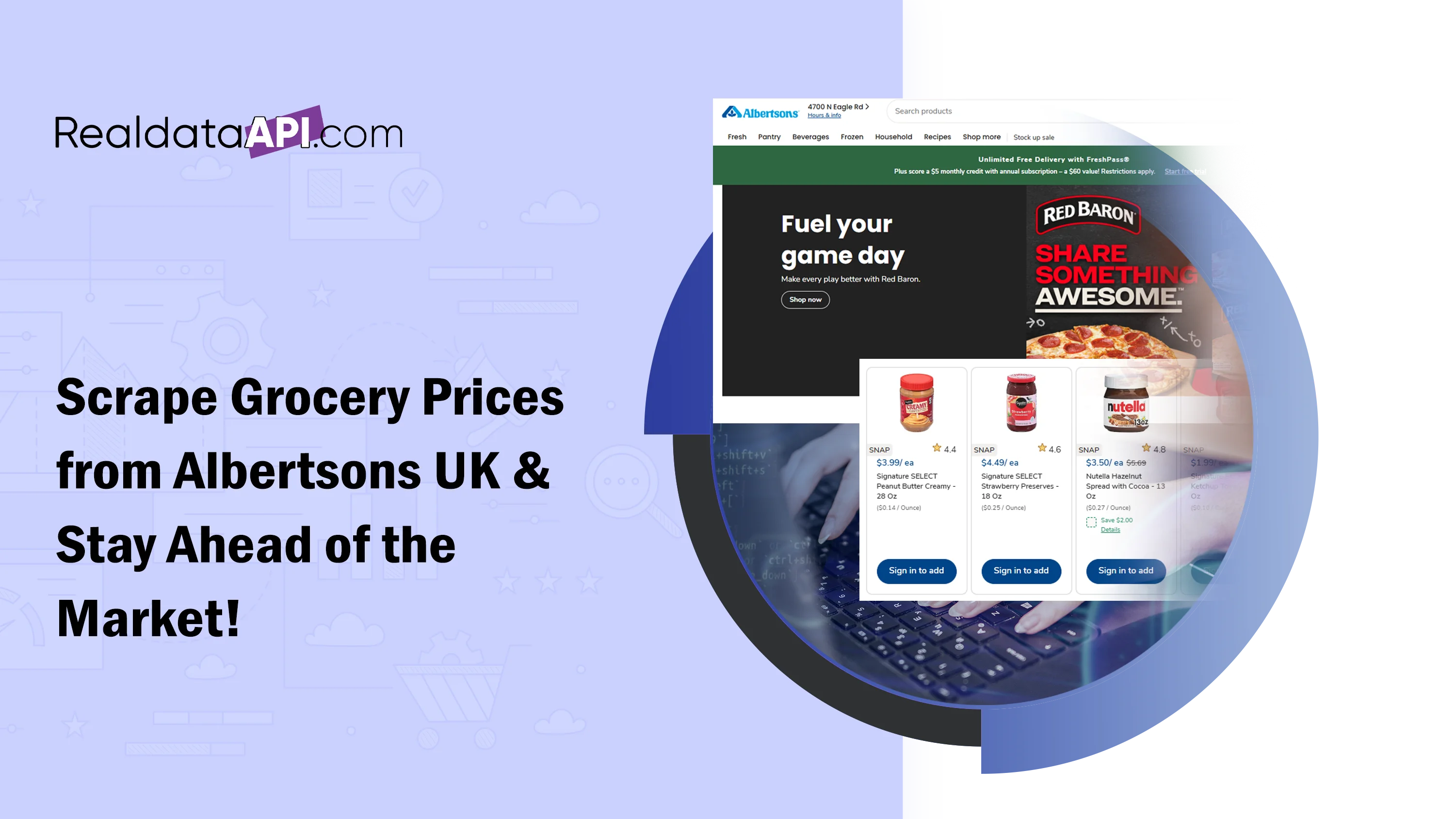
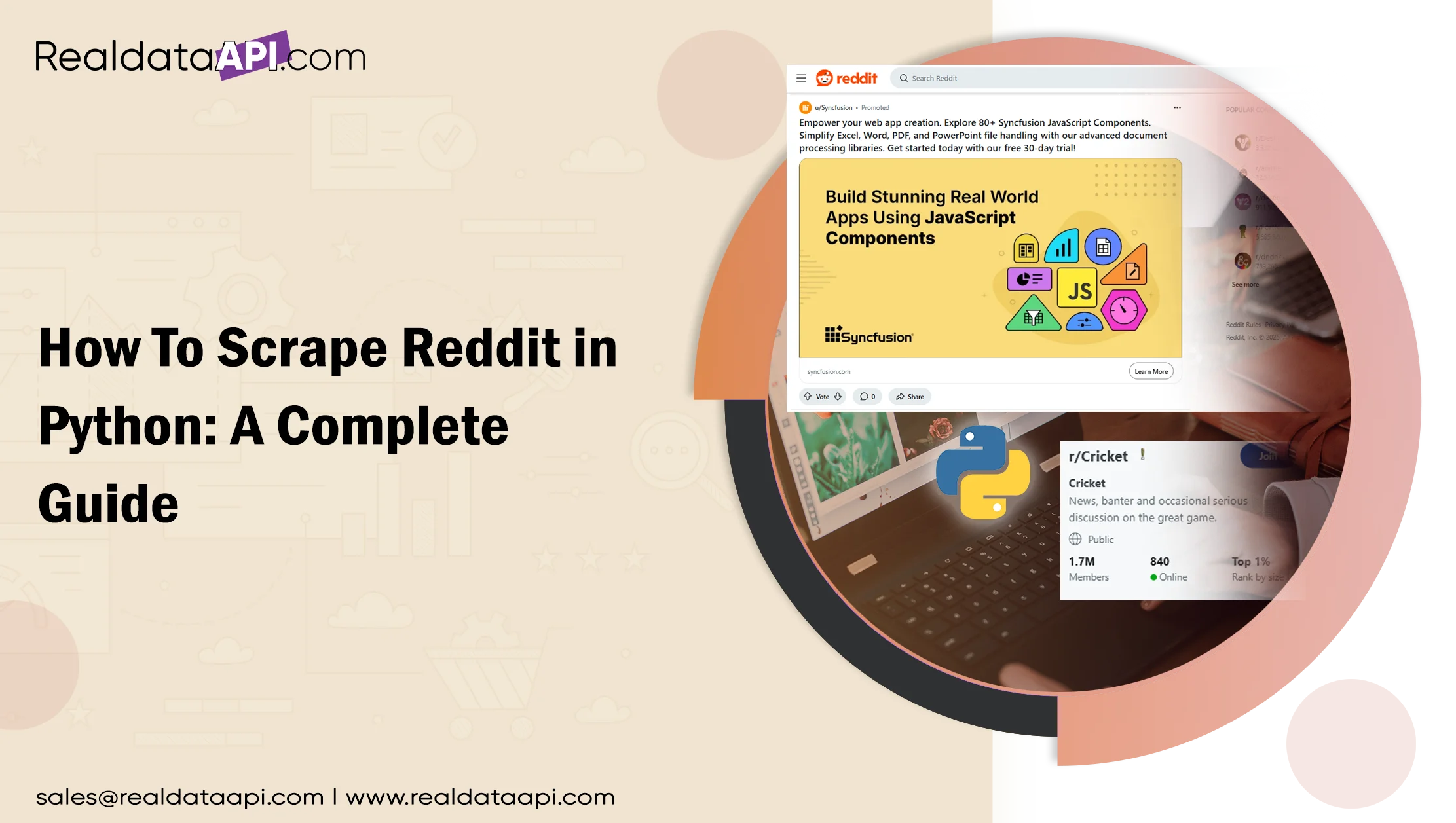
Introduction
Reddit is one of the most valuable sources of real-time discussions, opinions, and trends. Whether you're a researcher, marketer, or developer, extracting data from Reddit can provide powerful insights. But how do you scrape Reddit pages effectively? Should you use the Reddit Scraper or go for Reddit Scraping?In this guide, we will explore different methods of extracting data from Reddit using Python, compare Reddit API vs. Reddit Scraping, and show you how to use web scraping with Python to gather Reddit data.
Why Scrape Reddit?
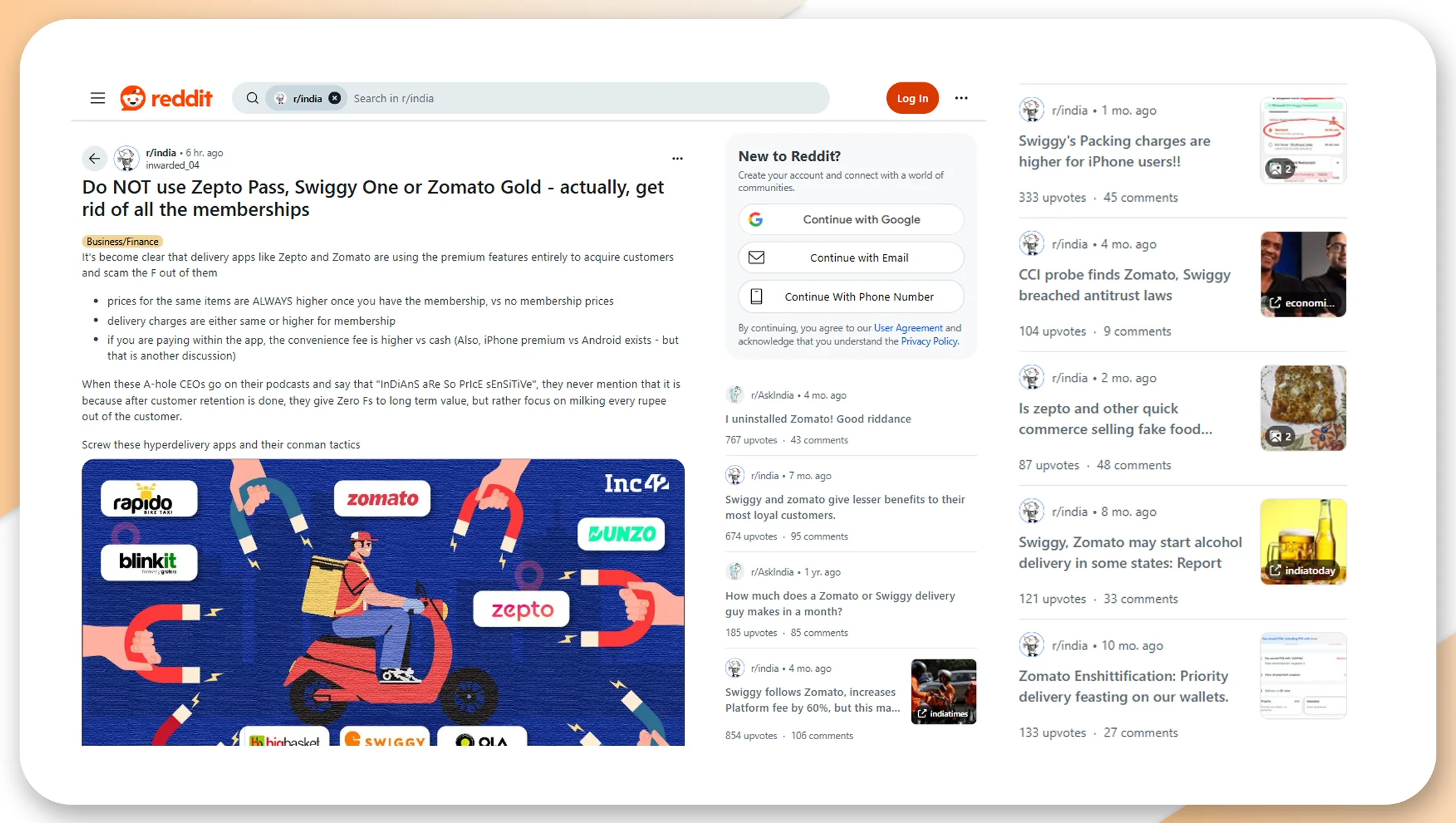
Scraping Reddit allows you to:
- Analyze trending discussions in your niche
- Monitor brand mentions and public sentiment
- Gather data for AI and machine learning models
- Extract pricing, product reviews, and competitive insights
But before we start, let’s understand the two main approaches to extract Reddit data:
Reddit API vs. Reddit Scraping: Which One to Choose?
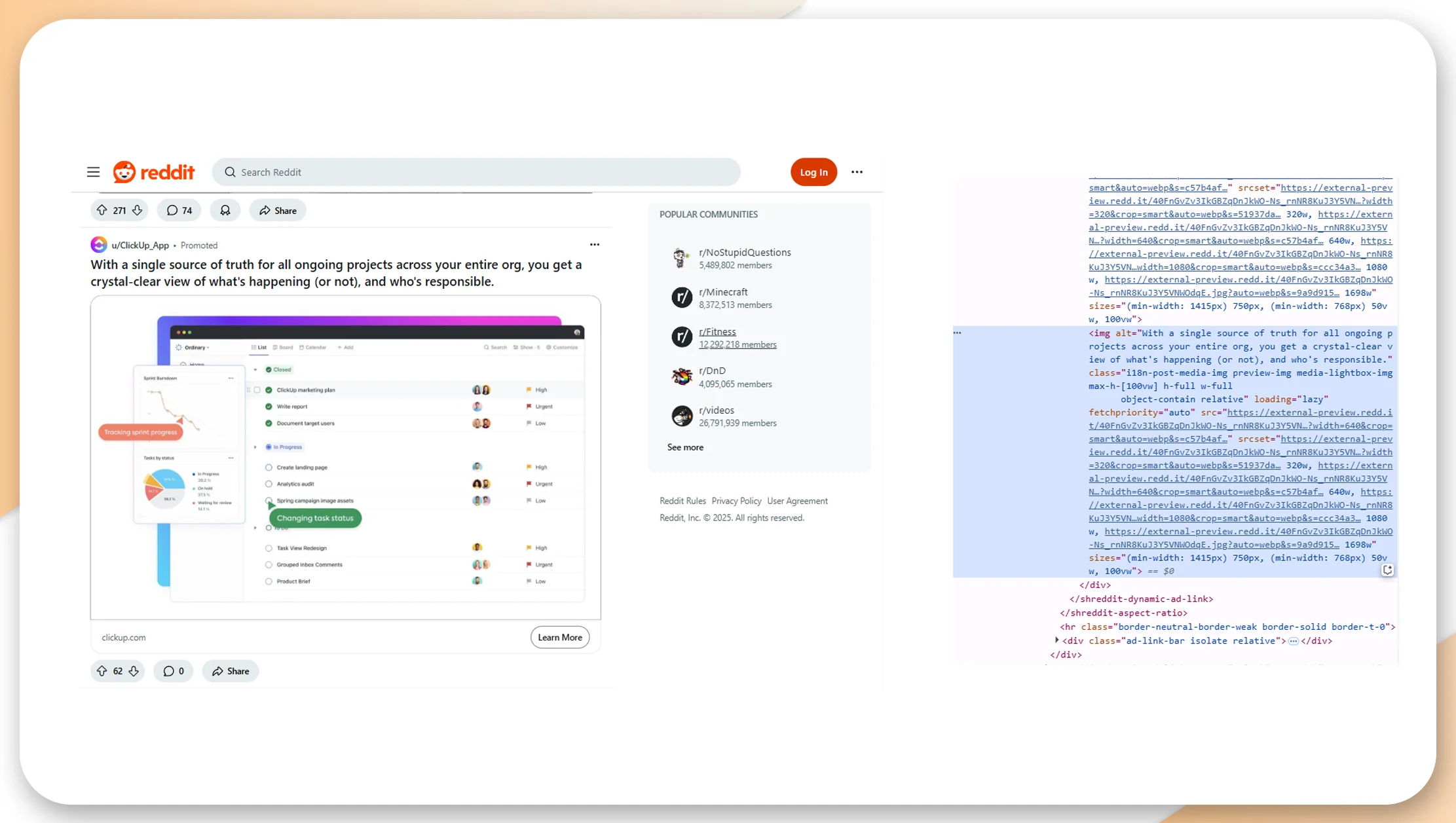
1. Reddit API
Reddit offers an official API that allows developers to fetch data programmatically. However, it has some limitations:
Pros:
- Provides structured data in JSON format
- Complies with Reddit’s terms of service
- No risk of getting blocked
Cons:
- Limited data access (e.g., no access to deleted comments or private communities)
- API rate limits may slow down large-scale scraping
- Requires authentication with API keys
2. Reddit Scraping (Web Scraping with Python)
Instead of using the API, you can extract Reddit data by scraping the web pages directly.
Pros:
- No API restrictions or rate limits
- Access to full content, including deleted comments and user-generated posts
- Suitable for large-scale data extraction
Cons:
- Risk of getting blocked without proper techniques (e.g., proxies, headers)
- HTML structure changes may break your scraper
- Some subreddits restrict bot access
How to Scrape Reddit Page with Python?
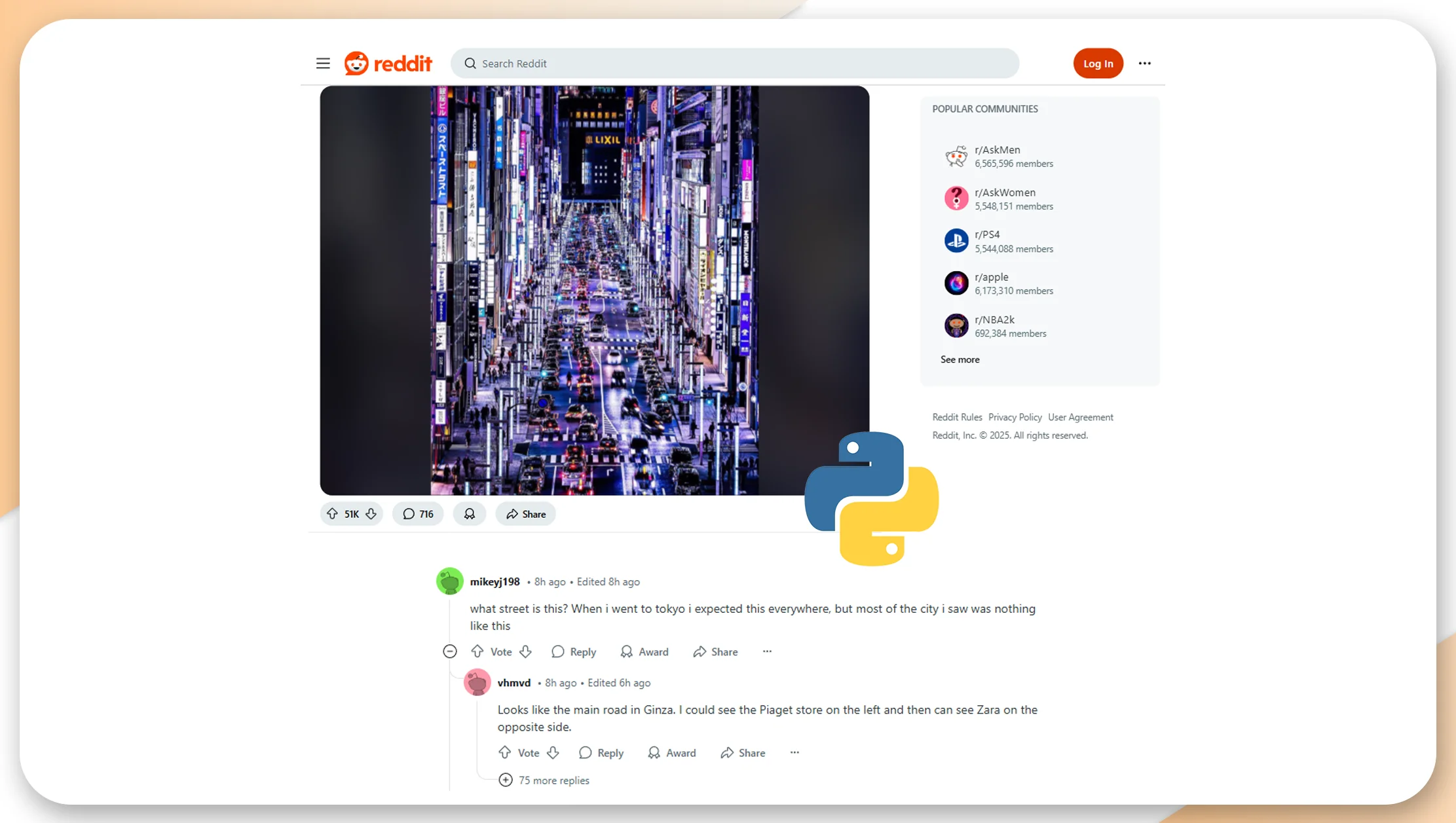
To scrape Reddit pages efficiently, we will use Python and the BeautifulSoup and Selenium libraries.
Prerequisites
Before we begin, install the required libraries:
pip install requests beautifulsoup4 selenium pandas
Method 1: Scraping Reddit Using the API
If you prefer using the official Reddit API, follow these steps:
Step 1: Register for Reddit API Credentials
1. Go to Reddit Developer Portal
2. Click on Create App
3. Fill in details and note down the Client ID and Client Secret
Step 2: Fetch Data Using PRAW (Python Reddit API Wrapper)
import praw
# Reddit API Credentials
reddit = praw.Reddit(
client_id="your_client_id",
client_secret="your_client_secret",
user_agent="your_user_agent"
)
# Fetch Top Posts from a Subreddit
subreddit = reddit.subreddit("technology")
for post in subreddit.hot(limit=5):
print(post.title, post.score, post.url)
Advantages: Fast, structured data, compliant with Reddit's policies
Disadvantages: Limited to API constraints
Method 2: Scraping Reddit Using BeautifulSoup
If you need to scrape Reddit without API limitations, you can use BeautifulSoup.
Step 1: Fetch and Parse Reddit HTML
import requests
from bs4 import BeautifulSoup
# Define Reddit URL
url = "https://www.reddit.com/r/technology/hot/"
# Set Headers to Avoid Blocks
headers = {"User-Agent": "Mozilla/5.0"}
response = requests.get(url, headers=headers)
# Parse HTML Content
soup = BeautifulSoup(response.text, "html.parser")
posts = soup.find_all("h3")
# Extract Titles
for post in posts[:5]:
print(post.text)
Advantages: No API limitations, works for all subreddits
Disadvantages: Prone to HTML structure changes, might get blocked
Method 3: Scraping Reddit Using Selenium (For Dynamic Content)
Some Reddit pages use JavaScript to load content dynamically. In such cases, use Selenium.
Step 1: Install and Setup Selenium
pip install selenium webdriver-manager
Step 2: Extract Reddit Posts Using Selenium
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
# Set Up Selenium WebDriver
options = webdriver.ChromeOptions()
options.add_argument("--headless")
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()), options=options)
# Open Reddit
driver.get("https://www.reddit.com/r/technology/hot/")
titles = driver.find_elements("css selector", "h3")
# Print Post Titles
for title in titles[:5]:
print(title.text)
# Close Browser
driver.quit()
Advantages: Works for JavaScript-rendered pages
Disadvantages: Slower than BeautifulSoup
Best Practices for Scraping Reddit Pages Safely
- Use Headers & User-Agent: Reddit can block scrapers without proper headers
- Rotate Proxies & IPs: Avoid detection by changing IP addresses
- Respect Robots.txt: Follow website policies
- Use API When Possible: The Reddit API is a safer and legal alternative
Conclusion
In this guide, we explored how to scrape Reddit pages using both the Reddit API and Reddit Scraping with Python.
- Use Reddit API if you need structured data and compliance
- Use BeautifulSoup for static HTML scraping
- Use Selenium for JavaScript-heavy pages
For large-scale data extraction, consider using Rotating Proxies and User-Agent Spoofing to avoid being blocked.
Need automated Reddit Scraping APIs? Contact Real Data API today!